A quick and dirty timer example. If you need to execute after a period of time use Thread.Timer(). This example uses simple thread and event to start and stop a clock in a Gtk 3 window counting up time in a label.
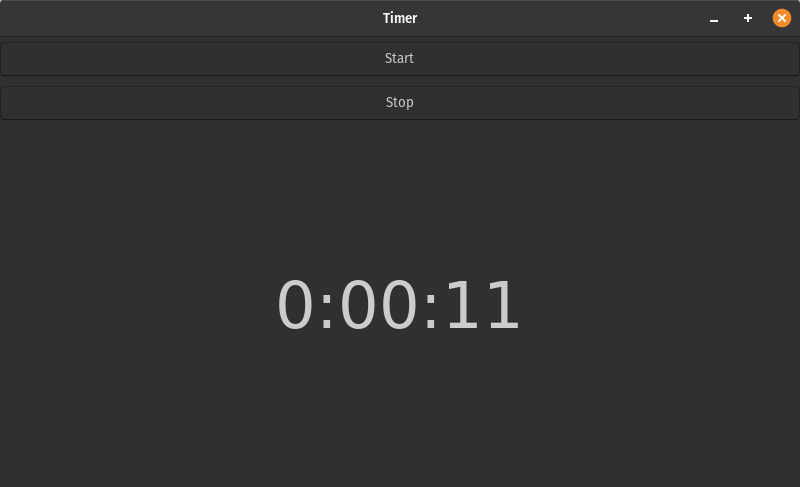
Python
x
61
61
1
import gi
2
gi.require_version('Gtk', '3.0')
3
from gi.repository import Gtk,Pango,GObject
4
import threading
5
import datetime
6
import time
7
8
class MyWindow(Gtk.Window):
9
10
def __init__(self):
11
self.timer = None
12
self.event = None
13
self.clock = '00:00:00'
14
15
Gtk.Window.__init__(self, title="Timer")
16
self.set_default_size(800, 450)
17
18
self.button_start = Gtk.Button(label="Start")
19
self.button_start.connect("clicked",self.start_timer)
20
21
self.button_stop = Gtk.Button(label="Stop")
22
self.button_stop.connect("clicked",self.stop_timer)
23
24
self.status = Gtk.Label()
25
self.status.set_text(self.clock)
26
# override_font is deprecated but good enough for a preview.
27
font = Pango.FontDescription("Tahoma 48")
28
self.status.override_font(font)
29
30
self.vbox = Gtk.VBox()
31
32
self.vbox.pack_start(self.button_start,False,False,5)
33
self.vbox.pack_start(self.button_stop,False,False,5)
34
self.vbox.pack_end(self.status,True,True,5)
35
36
self.add(self.vbox)
37
38
def get_time(self):
39
seconds = 0
40
while not self.event.is_set():
41
seconds += 1
42
self.clock = str(datetime.timedelta(seconds = seconds))
43
self.status.set_text(self.clock)
44
time.sleep(1)
45
46
def start_timer(self,button):
47
print('start')
48
self.timer = threading.Thread(target=self.get_time)
49
self.event = threading.Event()
50
self.timer.daemon=True
51
self.timer.start()
52
53
def stop_timer(self,button):
54
print('stop')
55
self.event.set()
56
self.timer = None
57
58
win = MyWindow()
59
win.connect("destroy", Gtk.main_quit)
60
win.show_all()
61
Gtk.main()